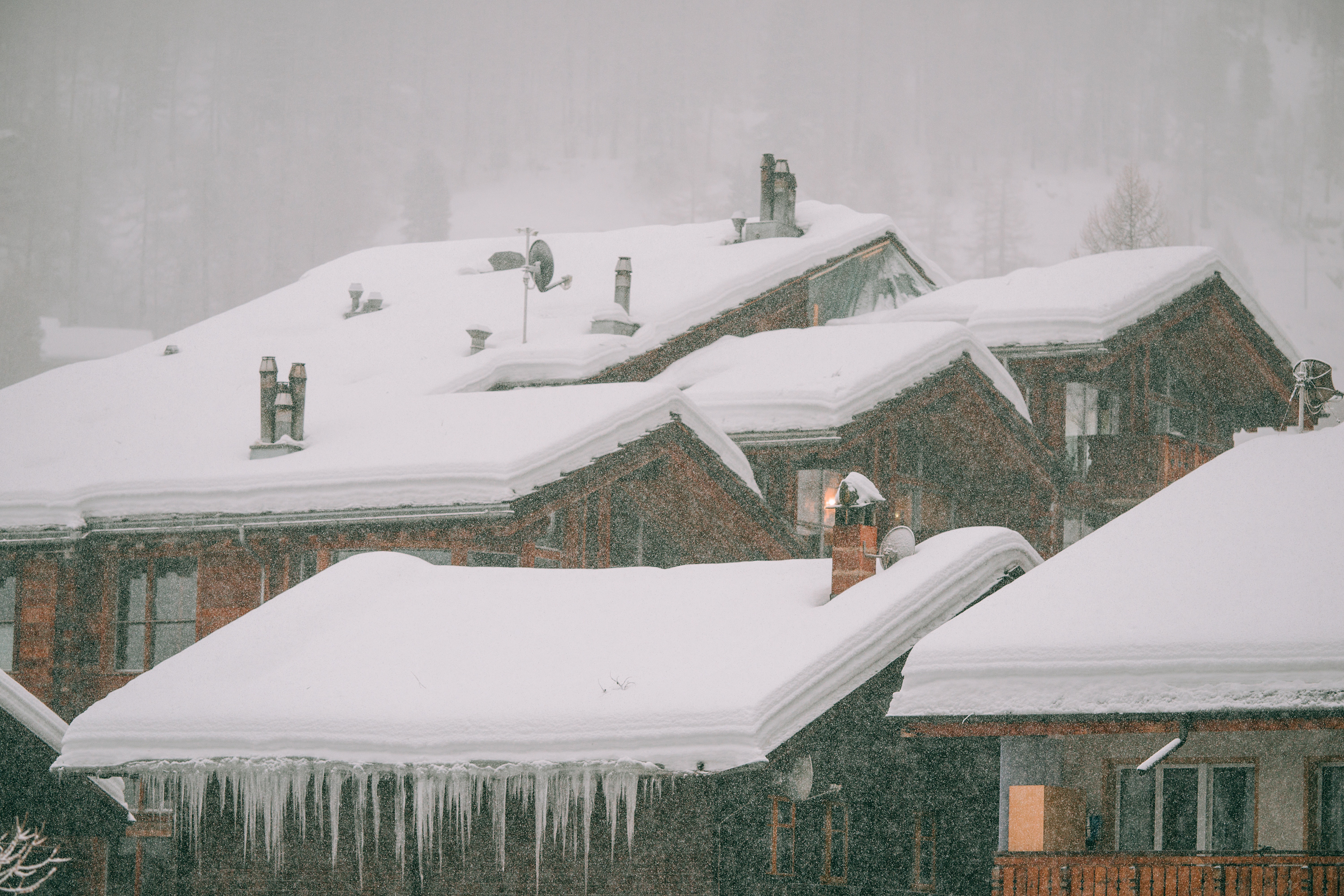
Numpy笔记
七月 15, 2021
3446
Numpy学习笔记
资料来源:Python知识手册,csdn
- 常用list和tuple来创建ndarray数据类型
1 |
|
创建时,可以指定数值类型
1 |
|
- reshape函数:将ndarray塑性
1 |
|
- 属性
ndim输出ndarray的维度属性(行数),shape输出尺度属性
size属性保存ndarray中元素的数量
flat属性,返回一个numpy.flatiter的可迭代对象(亦可索引,赋值)
1 |
|
- ndarray可进行切片和索引,一维与list类似
二维情况如图
1 |
|
逗号前竖着索引,逗号后横着索引
- array的叠加
水平叠加
1 |
|
垂直叠加
1 |
|
自定义方向
1 |
|
- array的拆分
split函数 - 类型转换
数组转列表,用tolist函数
1 |
|
将内容转化为指定类型
1 |
|
- 常用统计函数
sum()求和
mean()均值
ptp()沿某个轴返回最大值减最小值
std()标准偏差
var()方差
cumsum()累加值
comprod()累乘值 - random.rand函数
给定维度生成[0,1)间的ndarray
1 |
|
random.randn函数
返回一组给定维度的具有正态分布的ndarrayrandom.randint(low, high=None, size=None, dtype=’l’)函数
返回随机整数, low为下限,high为上限,size是维度,dtype是数据类型生成随即小数的四种方法
random.random_sample
random.random
random.ranf
random.samplerandom.choice(a, size=None, replace=True, p=None)函数
a表示数据的取值范围是0到a
size对应数组的维度
p是出现的概率
1 |
|
- Meshgrid函数
若向量x为一行m列,y为一行n列。经过mesgrid函数变换之后,产生了对应的X,Y的维度是(n*m)。也就是说,最终结果都是(n·m),依据x,y的长度不同,复制方式也会不同。
1 |
|
补充:
flat函数:flat返回的是一个迭代器,可以用for访问数组每一个元素,不可打印。
1 |
|
zip函数:将相同大小的对象对应位置元素打包成元组
>>>a = [1,2,3]
>>> b = [4,5,6]
>>> c = [4,5,6,7,8]
>>> zipped = zip(a,b) # 打包为元组的列表
[(1, 4), (2, 5), (3, 6)]
>>> zip(a,c) # 元素个数与最短的列表一致
[(1, 4), (2, 5), (3, 6)]
>>> zip(*zipped) # 与 zip 相反,*zipped 可理解为解压,返回二维矩阵式
[(1, 2, 3), (4, 5, 6)]
- 本文作者:Guo tianyu
- 本文链接:https://guotianyu-2020.github.io/2021/07/15/Numpy%E7%AC%94%E8%AE%B0/index.html
- 版权声明:本博客所有文章均采用 BY-NC-SA 许可协议,转载请注明出处!
查看评论