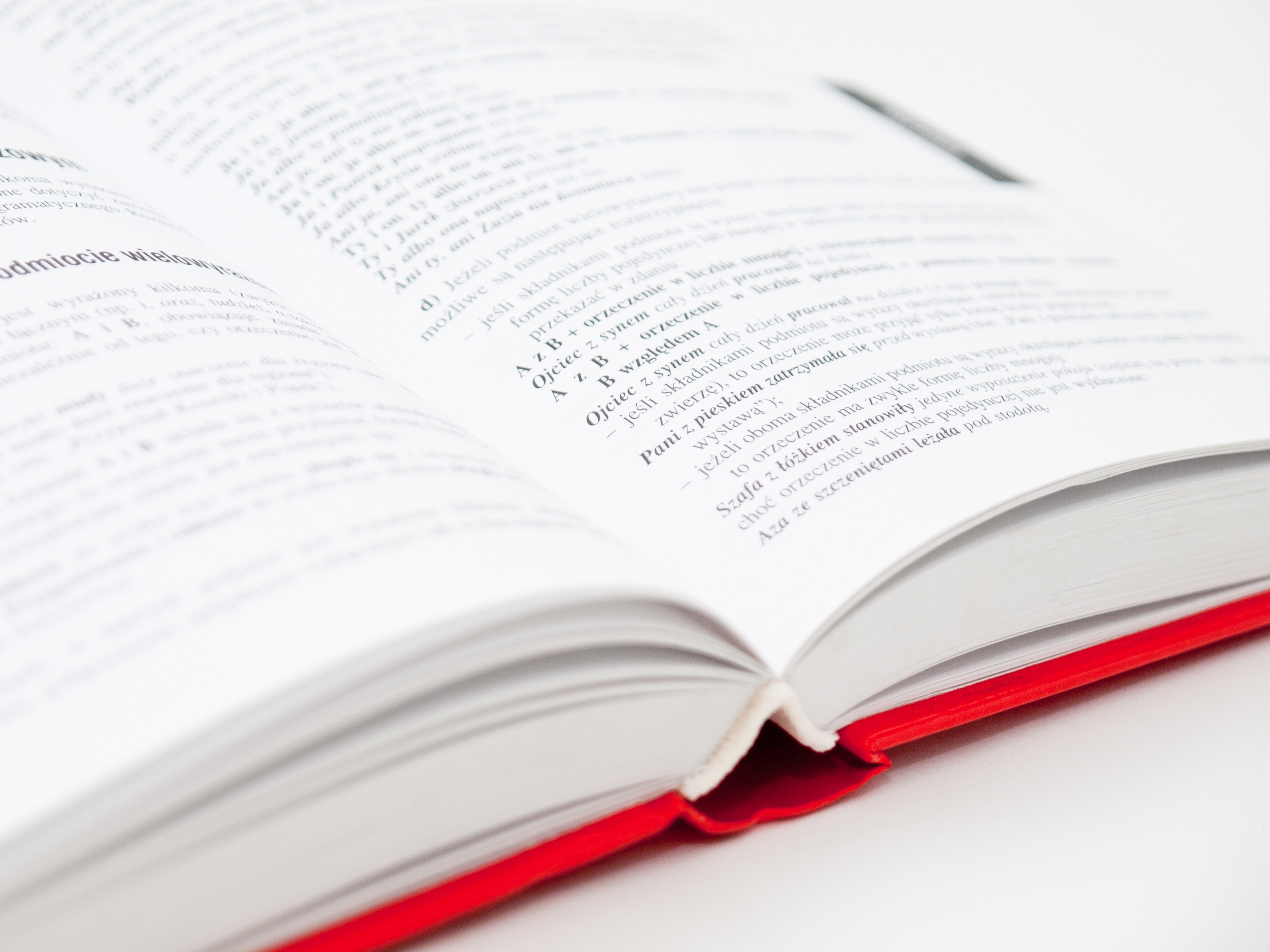
图的C语言实现
八月 28, 2021
6811
本文使用C语言完成图的创建(邻接矩阵和邻接表两种方法)与两种遍历方式(深度优先:DFS与广度优先:BFS)。
1.代码部分
1.1图的创建
(1)用邻接矩阵创建
结构体定义:
1 |
|
分别使用一维数组vex创建顶点集,二维数组arc创建边集(二维数组的x,y存放边的两个点,数值本身存放权值)
传入AMGraph指针开始创建。
1 |
|
其中的LocateVex函数定义如下:
1 |
|
(2)用邻接表创建
结构体定义:
1 |
|
VNode是每一个顶点对应的链表的头节点,Arcnode是其他节点。ALGraph是由头节点形成的线性表。
传入ALGraph指针开始创建:
1 |
|
其中LocateVex2函数:
1 |
|
1.2图的遍历
(1)深度优先算法(递归思想)
1 |
|
(2)广度优先算法
1 |
|
其中用到的函数:
1 |
|
主函数部分:
1 |
|
2.改进与反思
不知何时free掉分配的内存。。
- 本文作者:Guo tianyu
- 本文链接:https://guotianyu-2020.github.io/2021/08/28/%E5%9B%BE%E7%9A%84C%E8%AF%AD%E8%A8%80%E5%AE%9E%E7%8E%B0/index.html
- 版权声明:本博客所有文章均采用 BY-NC-SA 许可协议,转载请注明出处!
查看评论